前言
本文章结合VUE核心技术-尚硅谷的11-17集做的归纳总结。
事件处理
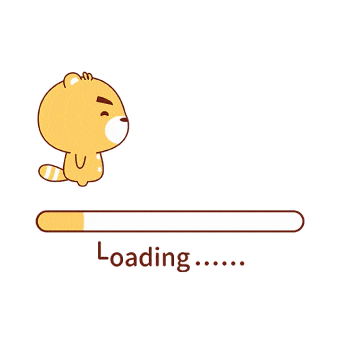
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| <body> <div id="demo"> <h2>1. 绑定监听</h2>
<button @click="test1">test1</button> <button @click="test2('test')">test2</button> <button @click="test3">test3</button> <button @click="test4(13,$event)">test4</button>
<h2>2. 事件修饰符</h2> <div style="width: 200px;height: 200px;background:red;" @click="test5"> <div style="width: 100px;height: 100px;background:blue;" @click.stop="test6"></div> </div>
<a href="https://www.baidu.com" @click.prevent="test7">去百度</a>
<h2>3. 按键修饰符</h2> <input type="text" @keyup="test8"> <br> <input type="text" @keyup.enter="test9"> </div> </body> <script>
vm = new Vue({ el:'#demo', methods:{ test1(){ alert('test'); },
test2(msg){ alert(msg); },
test3(event){ alert(event.target.innerHTML); },
test4(number,event){ alert(number + '---' + event.target.innerHTML); }, test5(){ alert('out'); }, test6(){ alert('inner'); }, test7(){ alert('点击了'); }, test8(event){ if(event.keyCode === 13){ alert(event.target.value + ' ' + event.keyCode); } }, test9(event){ alert(event.target.value + ' ' + event.keyCode); }, } }) </script>
|
体验一下
绑定监听
@click=""
可以再字符串中写入调用的函数名,通过加括号来获取参数
- 如果不传入参数,默认可以接受一个 $event 参数
事件修饰符
按键修饰符
@keyup=""
可以绑定键盘事件
- `@keyup.enter=””
可以实现enter键触发 代替
event.keyCode === 13` 的判断
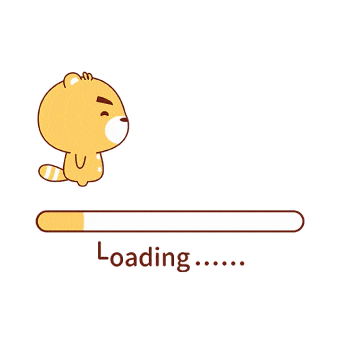
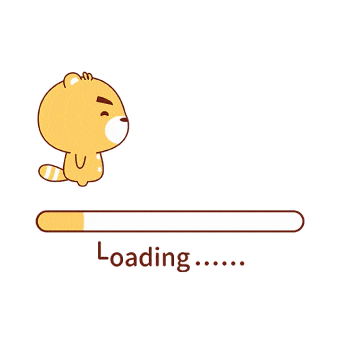
表单输入绑定
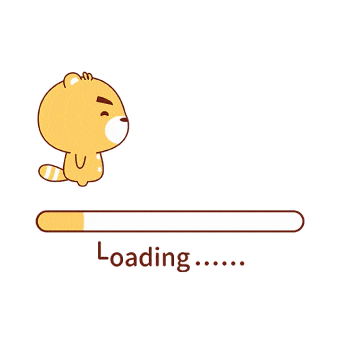
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| <body> <div id="demo"> <form action="/xxx" @submit="handleSumbit"> <span>用户名:</span> <input type="text" v-model="username"> <br> <span>密码:</span> <input type="password" v-model="pwd"> <br> <span>性别:</span> <input type="radio"id="female" value="女" v-model="sex"> <label for="female">女</label> <input type="radio"id="male" value="男" v-model="sex"> <label for="male">男</label> <br> <span>爱好:</span> <input type="checkbox"id="basket" value="basket" v-model="likes"> <label for="basket">篮球</label> <input type="checkbox"id="foot" value="foot" v-model="likes"> <label for="foot">足球</label> <input type="checkbox"id="pingpang" value="pingpang" v-model="likes"> <label for="pingpang">乒乓</label><br> <span>城市:</span> <select v-model="cityId"> <option value="">未选择</option> <option :value="city.id" v-for="(city,index) in allCitys" :key="index">{{city.name}}</option> </select> <br> <span>介绍:</span> <textarea rows="10" v-model="desc"></textarea> <br> <br> <input type="submit"value="注册"> </form> </div> </body> <script> vm = new Vue({ el:'#demo', data:{ username:'', pwd:'', sex:'男', likes:['foot'], allCitys:[{id:1,name:'北京'},{id:2,name:'上海'},{id:3,name:'广东'}], cityId:'3', desc:'' }, methods:{ handleSumbit(){ alert(this.username,this.pwd,this.likes,this.cityId,this.desc) } } }) </script>
|
体验一下
v-model 可以绑定到HTML的组件上,实现表单数据的抓取
生命周期
官网介绍
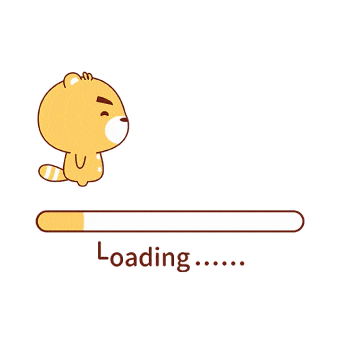
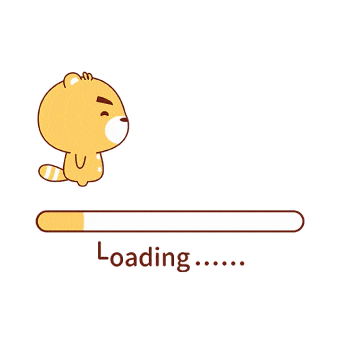
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <body> <div id="demo"> <button @click="destroyVM">destroy vm</button> <p v-show="isShow"> l0v0.com </p> </div> </body> <script> const vm = new Vue({ el:'#demo', data:{ isShow:true, }, mounted(){ this.internalId = setInterval(() => { this.isShow = !this.isShow }, 1000); }, beforeDestry(){ clearInterval(this.internalId) }, methods:{ destroyVM(){ this.$destroy() } }, })
</script>
|
体验一下
生命周期于 Android React 这些框架的生命周期大致相同
也就是 Vue 实例启动的时候回调用的函数
通过复写这些函数可以实现在对应的时间段执行对应的代码,确保实现效果符合预期。
过渡 & 动画
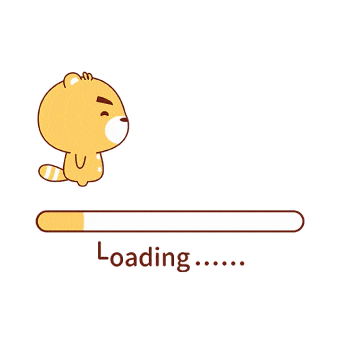
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73
| <style>
.xxx-enter-active, .xxx-leave-active{ transition: opacity 1s; }
.xxx-enter , .xxx-leave-to { opacity: 0; }
.move-enter-active{ transition: all 1s }
.move-leave-active{ transition: all 1s }
.move-enter, .move-leave-to{ opacity: 0; transform: translateX(20px); }
.bounce-enter-active { animation: bounce-in .5s; } .bounce-leave-active { animation: bounce-in .5s reverse; } @keyframes bounce-in { 0% { transform: scale(0); } 50% { transform: scale(1.5); } 100% { transform: scale(1); } }
</style> <body> <div id="demo" style="text-align: center"> <button @click="isShow=!isShow">toggle</button> <transition name="xxx"> <p v-show="isShow" class="xxx-enter-to">hello</p> </transition> <br> <button @click="isShow=!isShow">toggle</button> <transition name="move"> <p v-show="isShow" class="xxx-enter-to">hello</p> </transition> <br> <button @click="isShow = !isShow">Toggle show</button> <transition name="bounce"> <p v-if="isShow">Lorem ipsum dolor sit amet.</p> </transition> </div> </body> <script> const vm = new Vue({ el:'#demo', data:{ isShow:true, }, })
</script>
|
体验一下
通过 transition 标签来定义相关CSS快速实现过渡效果 官网参考
过滤器
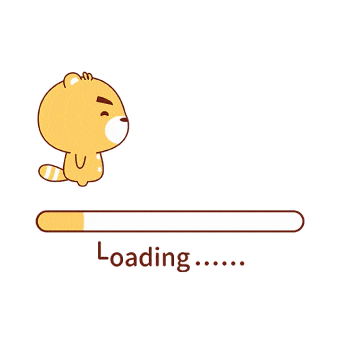
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <body> <div id="demo"> <h2>显示格式化的日期时间</h2> <p>{{date}}</p> <p>完整版 : {{date | dateString}} </p> <p>年月日 : {{date | dateString('YYYY-MM-DD')}} </p> <p>时分秒 : {{date | dateString('HH:mm:ss')}} </p> </div> </body> <script>
Vue.filter('dateString',function(value,format = 'YYYY-MM-DD HH:mm:ss'){ return moment(value).format(format) })
const vm = new Vue({ el:'#demo', data:{ date:new Date(), }, })
</script>
|
体验一下
通过 moment.js 对时间进行格式化
通过过滤器可以给定特定条件返回对应的值
过滤器
常用内置指令
- v:text:更新元素的 textContent
- V-htmL:更新元素的 innerHTML
- v-if:如果为true,当前标签才会输出到页面
- v-else:如果为false,当前标签才会输出到页面
- v-show:通过控制dispLay样式来控制显示/隐藏
- V-for:遍历数组/对象
- V-on:绑定事件监听,一般简写为@
- v-bind:强制定解析表达式,可以省略v-bind
- V-modeL:双向数据绑定
- ref:为某个元素注册一个唯一标识,vue对象通过sels属性访问这个元素对象
- v-cLoak:使用它防止闪现表达式,与css配合:[v-cloak]{display:none}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <style> [v-cloak] { display: none; } </style>
<body> <div id="demo"> <p>l0v0.com</p> <button @click="hint">提示</button> <p>{{msg}}</p> <p v-cloak>{{msg}}</p> </div> </body> <script> const vm = new Vue({ el:'#demo', data:{ msg: 'l0v0', }, methods:{ hint(){ alert(this.$refs.content.textContent) } } })
</script>
|
体验一下
自定义指令
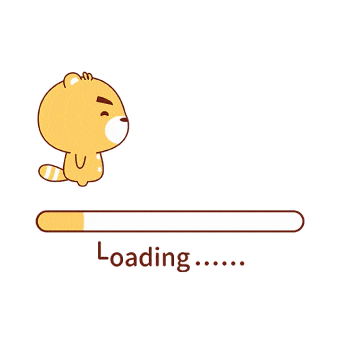
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| <body>
<div id="demo"> <p v-upper-text='msg1'></p> <p v-lower-text='msg1'></p> </div> <div id="test"> <p v-upper-text='msg1'></p> <p v-lower-text='msg1'></p> </div> </body> <script>
Vue.directive('upper-text',function(el,binding){ el.innerHTML=binding.value.toUpperCase() })
new Vue({ el:'#demo', data:{ msg1: 'l0v0', }, directives:{ 'lower-text': function(el,binding){ el.textContent = binding.value.toLowerCase() } }, methods:{ hint(){ alert(this.$refs.content.textContent) } } })
new Vue({ el:'#test', data:{ msg1: 'l0v0', }, })
</script>
|
体验一下
- 注册指令 - 在Vue实例加入 directives 属性进行注册 - 注册会返回绑定的element
- 使用指令 - 在绑定的属性上加上 v- 就可以和内置指令一样使用特殊效果的属性
插件
官网参考
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| (function () { const MyPlugin = {} MyPlugin.install = function (Vue, options) { Vue.myGlobalMethod = function () { };
Vue.directive("my-directive", function (el, binding) { el.textContent = binding.value.toUpperCase(); })
Vue.prototype.$myMethod = function (methodOptions) { }; };
window.MyPlugin = MyPlugin; })();
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <script src="./14.js"></script> <body> <div id="demo"> <p v-my-directive="msg"></p> </div> </body> <script>
Vue.use(MyPlugin)
const vm = new Vue({ el: '#demo', data: { msg: 'l0v0', } }) </script>
|
体验一下
编写 Vue 插件步骤
- Vue.js 的插件应该暴露一个 install 方法。这个方法的第一个参数是 Vue 构造器,第二个参数是一个可选的选项对象
- 添加全局方法或属性
- 添加全局资源
- 注入组件选项
- 添加实例方法